sqla-filters: Nodes¶
Base nodes¶
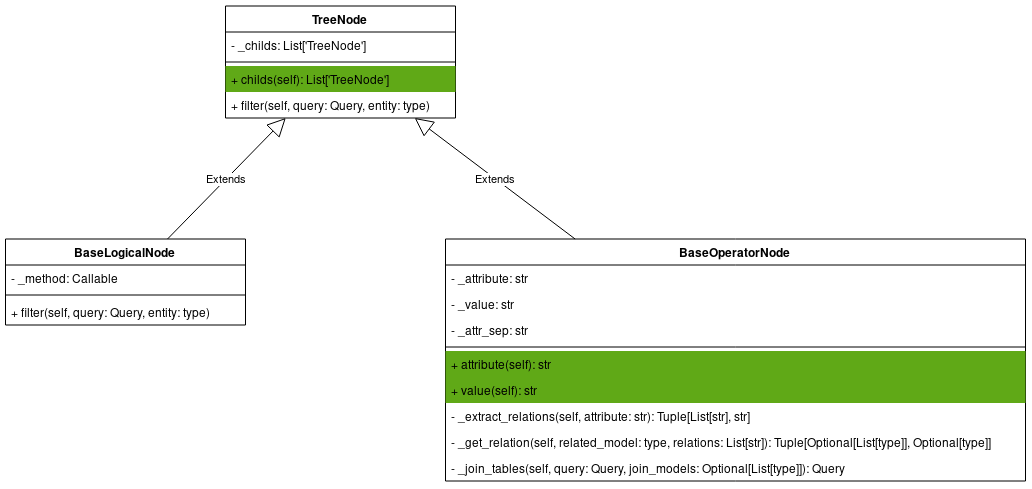
Tree¶
-
class
sqla_filters.nodes.base.
TreeNode
[source]¶ Abstract base class for every nodes.
This class
-
childs
¶ Property that return the node childs list.
Returns: The node childs list. Return type: List[TreeNode]
-
filter
(query: sqlalchemy.orm.query.Query, entity: type) → Tuple[sqlalchemy.orm.query.Query, Any][source]¶ Define the filter function that every node must to implement.
Parameters: - query (Query) – The sqlalchemy query.
- entity (type) – The entity model.
Returns: The filtered query.
Return type: Tuple[Query, Any]
-
Base logical¶
-
class
sqla_filters.nodes.base.
BaseLogicalNode
(*args, method=<function default_method>, **kwargs)[source]¶ -
filter
(query: sqlalchemy.orm.query.Query, entity: type) → Tuple[sqlalchemy.orm.query.Query, Any][source]¶ Apply the _method to all childs of the node.
Parameters: - query (Query) – The sqlachemy query.
- entity (type) – The entity model of the query.
Returns: A tuple with in first place the updated query and in second place the list of filters to apply to the query.
Return type: Tuple[Query, Any]
-
Base operational¶
-
class
sqla_filters.nodes.base.
BaseOperationalNode
(attribute: str, value: Any, attr_sep: str = '.')[source]¶ -
_extract_relations
(attribute: str) → Tuple[List[str], str][source]¶ Split and return the list of relation(s) and the attribute.
Parameters: attribute (str) – Returns: A tuple where the first element is the list of related entities and the second is the attribute. Return type: Tuple[List[str], str]
-
_get_relation
(related_model: type, relations: List[str]) → Tuple[Optional[List[type]], Optional[type]][source]¶ Transform the list of relation to list of class.
Parameters: - related_mode (type) – The model of the query.
- relations (List[str]) – The relation list get from the _extract_relations.
Returns: Tuple with the list of relations (class) and the second element is the last relation class.
Return type: Tuple[Optional[List[type]], Optional[type]]
-
_join_tables
(query: sqlalchemy.orm.query.Query, join_models: Optional[List[type]]) → sqlalchemy.orm.query.Query[source]¶ Method to make the join when relation is found.
Parameters: - query (Query) – The sqlalchemy query.
- join_models (Optional[List[type]]) – The list of joined models get from the method _get_relation.
Returns: The new Query with the joined tables.
Return type: Query
-
attribute
¶ Property that return the model attribute.
Returns: The model attribute. Return type: str
-
filter
(query: sqlalchemy.orm.query.Query, entity: type) → Tuple[sqlalchemy.orm.query.Query, Any][source]¶ Add a filters to the list of filters to apply.
Warning
This method must be override in childs nodes.
Parameters: - query (Query) – The sqlachemy query.
- entity (type) – The entity model of the query.
Returns: A tuple with in first place the updated query and in second place the list of filters to apply to the query.
Return type: Tuple[Query, Any]
-
value
¶ Property that return the value of the model attribute.
Returns: The value of the model attribute. Return type: Any
-
Operational nodes¶
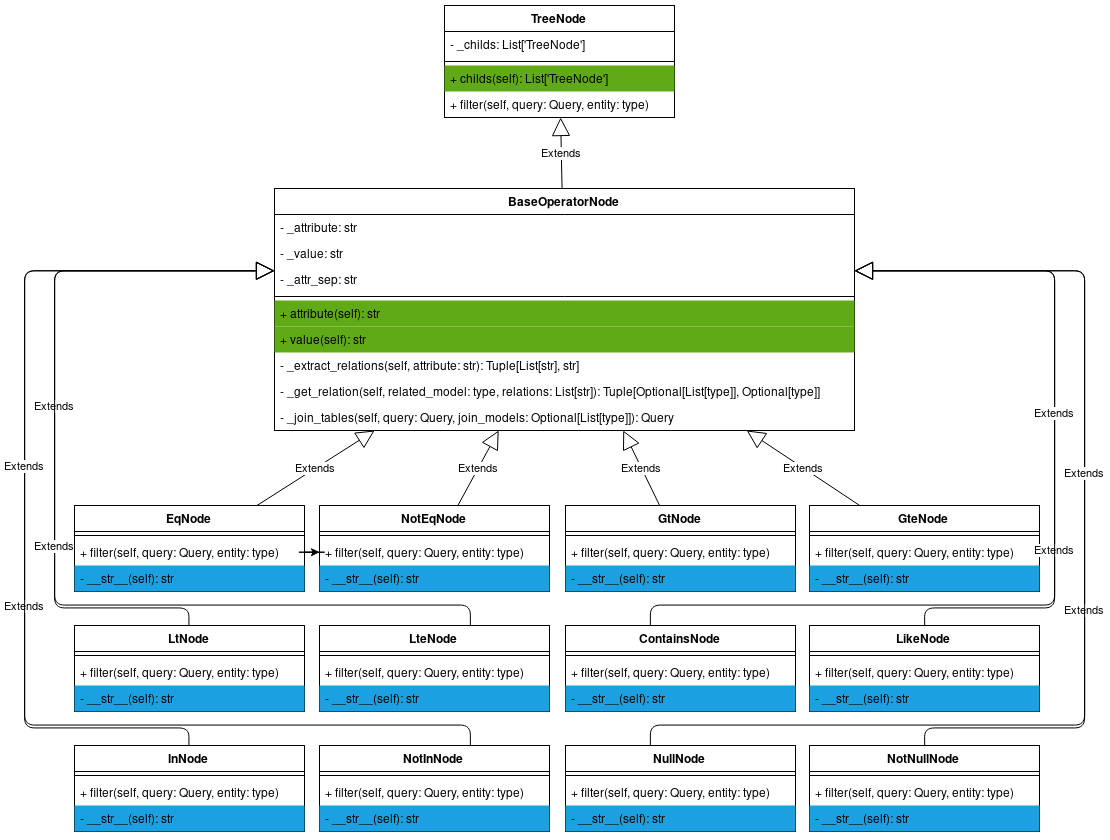
Equality¶
Greater / Greater equal¶
Lower / Lower equal¶
Contains¶
Like¶
In¶
-
class
sqla_filters.nodes.operational.
InNode
(attribute: str, value: Any, attr_sep: str = '.')[source]¶ InNode class.
This node test if an attribut is in a list of values. This function have the behavior of the in in the sql language. This node use the attr.in function of a model attribute.
-
class
sqla_filters.nodes.operational.
NotInNode
(attribute: str, value: Any, attr_sep: str = '.')[source]¶ NotInNode class.
This node test if an attribut is not in a list of values. This function have the behavior of the not in in the sql language. This node use the ~attr.in_ function of a model attribute.
Null¶
-
class
sqla_filters.nodes.operational.
InNode
(attribute: str, value: Any, attr_sep: str = '.')[source] InNode class.
This node test if an attribut is in a list of values. This function have the behavior of the in in the sql language. This node use the attr.in function of a model attribute.
-
class
sqla_filters.nodes.operational.
NotInNode
(attribute: str, value: Any, attr_sep: str = '.')[source] NotInNode class.
This node test if an attribut is not in a list of values. This function have the behavior of the not in in the sql language. This node use the ~attr.in_ function of a model attribute.